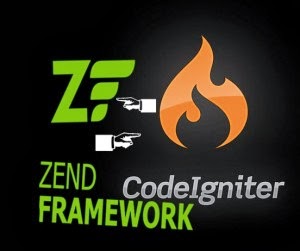
First you need to download Zend libraries (Zend Framework 1.12.3 Minimal) from their official site.
Then copy the library folder to “Application/Libraries/” folder
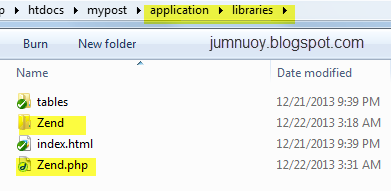
Now you need to create a loader for codeigniter to use those libraries. Save this code to Application/Libraries/Zend.php file as above
if (!defined('BASEPATH')) { exit('No direct script access allowed'); }
class Zend {
public function __construct($class = NULL) {
ini_set('include_path',ini_get('include_path') . PATH_SEPARATOR . APPPATH . 'libraries');
if ($class) {
require_once (string) $class . EXT;
log_message('debug', "Zend Class $class Loaded");
}else {
log_message('debug', "Zend Class Initialized");
}
}
public function load($sClassName) {
require_once (string) $sClassName . EXT;
log_message('debug', "-> Zend Class $sClassName Loaded from the library");
}
}
That’s it. Now call library what you need from your method in Controller.
Here I show one example to load Blogger class.
$this->load->library('zend');
$this->zend->load('Zend/Loader');
Zend_Loader::loadClass('Zend_Gdata');
Zend_Loader::loadClass('Zend_Gdata_Query');
Zend_Loader::loadClass('Zend_Gdata_ClientLogin');
Here I show one example to load Google Spreedsheet.
public function index(){
$this->load->library('zend');
$this->zend->load('Zend/Gdata/Spreadsheets');
$oSpreadSheet = new Zend_Gdata_Spreadsheets();
$entry = $oSpreadSheet->newCellEntry();
$cell = $oSpreadSheet->newCell();
$cell->setText('My cell value');
$cell->setRow('1');
$cell->setColumn('3');
$entry->cell = $cell;
var_dump( $entry );
}
Now enjoy the power of Zend in your Codeigniter web application.
Comments :
0 comments to “Integrate Zend framework library in codeigniter, Including Zend Library within Codeigniter”
Post a Comment